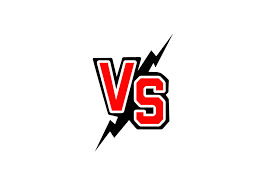
Hibernate CriteriaBuilder Vs QueryDSL
Hibernate Criteria and QueryDSL are two popular frameworks for creating and executing dynamic queries in Java. Both offer a way to programmatically build and execute queries, rather than writing them as static strings. In this article, we will compare and contrast the two frameworks, highlighting the key differences and similarities between them.
First, let’s briefly describe each framework. Hibernate Criteria is a framework that is part of the popular Hibernate ORM (Object-Relational Mapping) library. It allows developers to create dynamic, type-safe queries using a fluent API. This means that developers can chain together method calls to build up a query, rather than writing a query string. Hibernate Criteria also provides a way to specify query parameters using a variety of techniques, such as named parameters or positional parameters.
QueryDSL, on the other hand, is a standalone framework that is not tied to any particular ORM library. It is designed to be used with any data access layer, and provides a fluent API for creating and executing dynamic queries. Like Hibernate Criteria, QueryDSL allows developers to chain together method calls to build up a query, and provides a variety of ways to specify query parameters.
Now let’s compare the two frameworks. One key difference between Hibernate Criteria and QueryDSL is that Hibernate Criteria is tied to the Hibernate ORM library, while QueryDSL is a standalone framework that can be used with any data access layer. This means that if you are already using Hibernate in your project, Hibernate Criteria may be a natural choice. On the other hand, if you are using a different ORM library or no ORM at all, QueryDSL may be a better option.
Another difference is the way that the two frameworks handle type safety. Hibernate Criteria uses generics to provide type safety, which means that the type of the query result is checked at compile-time. QueryDSL, on the other hand, uses a technique called type-safe queries to provide type safety. This technique involves generating Java classes from a query definition, which are then used to build and execute the query. Both approaches provide type safety, but they do so in slightly different ways.
A final difference between the two frameworks is the way that they handle query parameters. Hibernate Criteria allows developers to specify query parameters using named parameters or positional parameters, while QueryDSL only supports named parameters. Both approaches have their advantages and disadvantages, and the choice of which to use may depend on the needs of your project.
In conclusion, Hibernate Criteria and QueryDSL are two popular frameworks for creating and executing dynamic queries in Java. Both offer a fluent API and support type safety, but Hibernate Criteria is tied to the Hibernate ORM library and supports both named and positional parameters, while QueryDSL is a standalone framework and only supports named parameters. Both frameworks have their strengths and weaknesses, and the choice of which to use will depend on the needs of your project.
Examples:
Let’s consider a simple example where we want to retrieve a list of users from a database, sorted by their last name in ascending order. Here is how we could do this using Hibernate Criteria:
CriteriaBuilder builder = session.getCriteriaBuilder();
CriteriaQuery<User> criteria = builder.createQuery(User.class);
Root<User> root = criteria.from(User.class);
criteria.select(root).orderBy(builder.asc(root.get("lastName")));
List<User> users = session.createQuery(criteria).getResultList();
And here is how we could do the same thing using QueryDSL:
QUser user = QUser.user;
List<User> users = queryFactory.selectFrom(user)
.orderBy(user.lastName.asc())
.fetch();
In both cases, we are creating a query that selects all users and orders them by the last name attribute in ascending order. The Hibernate Criteria example uses a fluent API to build up the query, while the QueryDSL example uses a DSL ( Domain Specific Language) to define the query. Both approaches allow us to create dynamic, type-safe queries without writing raw SQL.