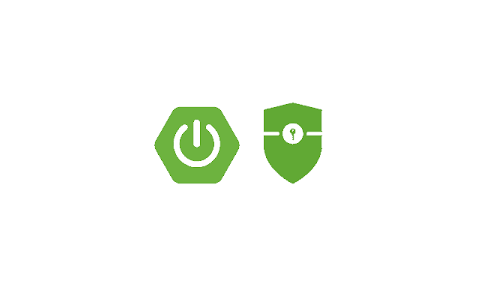
Spring Security and Spring Data
Spring is a popular Java-based framework for building web and enterprise applications. It offers a wide range of features and tools that make it easier to develop and maintain complex applications.
One of the key features of Spring is its support for authentication and authorization. Spring provides a number of tools and libraries to help developers build secure, authenticated applications. In this article, we’ll take a look at how to use Spring Security and Spring Data to build a simple, secure application with user authentication and authorization.
Spring Security
Spring Security is a powerful framework for building secure applications. It provides a number of features to help developers implement secure authentication and authorization in their applications, including:
Support for a wide range of authentication mechanisms, including LDAP, OAuth, and SAML Flexible configuration options to allow developers to customize the security of their application A number of built-in security patterns, such as role-based access control and password hashing To use Spring Security in your application, you’ll need to add the spring-security-web and spring-security-config dependencies to your project. These libraries provide the necessary classes and configurations to use Spring Security in your application.
Once you have these dependencies added to your project, you can start configuring Spring Security in your application. One way to do this is to create a WebSecurityConfigurerAdapter class, which allows you to define your security rules and configurations in a convenient, centralized location.
Here’s an example of how you might configure Spring Security in a WebSecurityConfigurerAdapter class:
Copy code @Configuration @EnableWebSecurity public class SecurityConfiguration extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(passwordEncoder());
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasAnyRole("USER", "ADMIN")
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
} In this example, we’re using a UserDetailsService to load user details from a database (more on this later). We’re also using a PasswordEncoder to hash and verify passwords when a user logs in.
We’re also defining some authorization rules using the antMatchers() method. These rules specify which URLs are protected by which roles. For example, the /admin/** URL is protected by the ADMIN role, while the /user/** URL is protected by either the USER or ADMIN role.
Finally, we’re using the formLogin() and logout() methods to configure the login and logout process for our application.
Spring Data Spring Data is a powerful framework for working with data in Java applications. It provides a number of libraries and tools for working with different types of data sources, including relational databases,
Guilherme Elizeire continue NoSQL databases, and even in-memory data stores.
One of the key features of Spring Data is its support for repositories. A repository is a pattern that allows you to abstract the details of how you store and retrieve data from a specific data source. This makes it easier to switch between different data sources without having to change the code that uses the data.
To use Spring Data in your application, you’ll need to add the appropriate dependencies for the data source you want to use. For example, if you want to use Spring Data with a MySQL database, you’ll need to add the spring-data-jpa dependency.
Once you have the appropriate dependencies added to your project, you can create a repository interface to define the methods you want to use to access and manipulate data in your application.
Here’s an example of a repository interface that uses Spring Data to access a MySQL database:
Copy code @Repository public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
} In this example, we’re creating a repository interface called UserRepository that extends the JpaRepository interface. The JpaRepository interface provides a number of methods for working with data in a JPA-compliant database, such as saving and retrieving entities.
We’re also defining a custom method called findByUsername() that allows us to search for a user by their username. This method will be automatically implemented by Spring Data based on the method name and the data source we’re using.
Tutorial Now that we’ve covered the basics of Spring Security and Spring Data, let’s walk through an example of how you might use these frameworks to build a simple, secure application with user authentication and authorization.
Prerequisites To follow along with this tutorial, you’ll need:
Java 8 or higher Maven 3 or higher A MySQL database Step 1: Set up the project Create a new Maven project and add the following dependencies to the pom.xml file:
Copy code
These dependencies will give you access to the core Spring Security and Spring Data libraries, as well as support for building web applications and connecting to a MySQL database.
Step 2: Configure the database Next, you’ll need to create a MySQL database and a user with access to that database. You can do this using the MySQL command line or a MySQL management tool like PHPMyAdmin.
Once you have your database set up, create a file called application.properties in
Guilherme Elizeire continue the src/main/resources directory and add the following configuration to connect to your database:
Copy code
spring.datasource.url=jdbc:mysql://localhost:3306/
Step 3: Create the user entity Next, we’ll create a simple User entity to represent a user in our application. Create a new Java class called User and add the following code:
Copy code @Entity public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false, unique = true)
private String username;
@Column(nullable = false)
private String password;
@Column(nullable = false)
private String role;
// getters and setters
} This entity has four fields: id, username, password, and role. The id field is the primary key for the entity and will be automatically generated by the database. The username and password fields will be used for authentication, and the role field will be used for authorization.
Step 4: Create the user service Next, we’ll create a service to handle user-related tasks, such as creating and updating users. Create a new Java interface called UserService and add the following code:
Copy code public interface UserService {
void createUser(User user);
void updateUser(User user);
User findByUsername(String username);
} This interface defines three methods: createUser(), updateUser(), and findByUsername(). We’ll implement these methods in the next step.
Step 5: Implement the user service Now we’ll create an implementation of the UserService interface. Create a new Java class called UserServiceImpl and add the following code:
Copy code @Service public class UserServiceImpl implements UserService {
@Autowired
private UserRepository userRepository;
@Override
public void createUser(User user) {
userRepository.save(user);
}
@Override
public void updateUser(User user) {
userRepository.save(user);
}
@Override
public User findByUsername(String username) {
return userRepository.findByUsername(username);
}
} This implementation uses the UserRepository interface (which we defined earlier) to access and manipulate data in the database. The save() method is provided by the JpaRepository interface and is used to create or update an entity in the database.
Step 6: Configure Spring Security Now we’ll configure Spring Security to use our UserService for authentication and authorization. Create a new Java class called SecurityConfiguration and add the following code:
Copy code @Configuration @EnableWebSecurity public class SecurityConfiguration extends WebSecurityConfigurerAdapter { @Autowired private UserService userService;
@Autowired private PasswordEncoder passwordEncoder;
@Override protected void configure(AuthenticationManagerBuilder auth) throws Exception { auth.userDetailsService(userDetailsService()).passwordEncoder(passwordEncoder); }
@Bean public PasswordEncoder passwordEncoder() { return new BCryptPasswordEncoder(); }
@Bean @Override public UserDetailsService userDetailsService() { return username -> { User user = userService.findByUsername(username); if (user == null) { throw new UsernameNotFoundException(username); } return new org.springframework.security.core.userdetails.User( user.getUsername(), user.getPassword(), AuthorityUtils.createAuthorityList(user.getRole()) ); }; }
@Override protected void configure(HttpSecurity http) throws Exception { http .authorizeRequests() .antMatchers(“/admin/”).hasRole(“ADMIN”) .antMatchers(“/user/”).hasAnyRole(“USER”, “ADMIN”) .anyRequest().authenticated() .and() .formLogin() .loginPage(“/login”) .permitAll() .and() .logout() .permitAll(); } }
Copy code
This configuration extends the WebSecurityConfigurerAdapter
class and overrides several of its methods to customize
the security of our application.
In the configure()
method, we’re using the userDetailsService()
method to specify that we want to use
our UserService
to load user details for authentication. We’re also specifying a PasswordEncoder
to hash and verify
passwords.
In the authorizeRequests()
method, we’re defining some authorization rules using the antMatchers()
method. These
rules specify which URLs are protected by which roles.
Finally, we’re using the formLogin()
and logout()
methods to configure the login and logout process for our
application.
Step 7: Create the login and logout controllers
Now we’ll create some simple controllers to handle the login and logout process. Create a new Java class
called LoginController
and add the following code:
@Controller
public class LoginController {
@GetMapping("/login")
public String login() {
return "login";
}
}
This controller has a single login() method that maps to the /login URL. When a user accesses this URL, they’ll see the login view.
Create a new Java class called LogoutController and add the following code:
Copy code @Controller public class LogoutController {
@GetMapping("/logout")
public String logout(HttpServletRequest request, HttpServletResponse response) {
Authentication auth = SecurityContextHolder.getContext().getAuthentication();
if (auth != null) {
new SecurityContextLogoutHandler().logout(request, response, auth);
}
return "redirect:/login?logout";
} } This controller has a single logout() method that maps to the /logout URL. When a user accesses this URL, they'll be logged out of the application and redirected to the login page.
Step 8: Create the views Finally, we’ll create some simple views for the login and logout process. Create a new file called login.html in the src/main/resources/templates directory and add the following code:
Copy code <!DOCTYPE html>
Login
This is a simple login form that allows a user to enter their username and password.
Create a new file called home.html in the src/main/resources/templates directory and add the following code:
Copy code <!DOCTYPE html>
Welcome!
You are logged in as
This is a simple home page that displays a welcome message and the username of the logged-in user. It also provides a link to log out of the application.
Step 9: Test the application Now that we’ve set up our application, let’s try it out! Start the application using the mvn spring-boot:run command, and then navigate to the /login URL in your web browser. You should see the login form.
Try logging in with a valid username and password. If everything is set up correctly, you should be redirected to the home page.
Try accessing the /admin URL. You should see an error message indicating that you don’t have the necessary permissions to access this URL.
Try logging out of the application by clicking the logout link on the home page. You should be redirected to the login page.
Congratulations, you’ve successfully set up a simple, secure application with user authentication and authorization using Spring Security and Spring Data!
Conclusion In this article, we’ve seen how to use Spring Security and Spring Data to build a simple, secure application with user authentication and authorization. We covered the basics of these frameworks and walked through an example of how to use them to build a working application.
There’s a lot more you can do with Spring Security and Spring Data, so be sure to explore their documentation to learn more about what they have to offer.