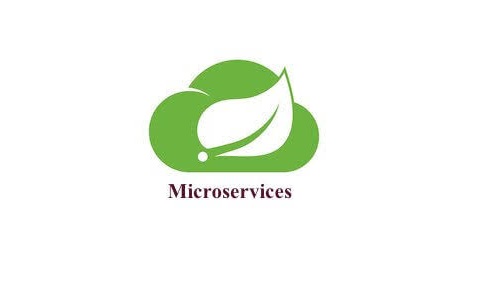
Microservices with Spring boot
Spring Boot is a popular framework for building standalone, production-grade Java applications. It provides a range of features that make it easier to develop and deploy applications, including support for microservices architecture.
Microservices architecture is a design approach that involves building a large application as a collection of small, independent services. Each service is responsible for a specific business capability and communicates with other services through well-defined interfaces. This allows for greater flexibility and scalability, as each service can be developed and deployed independently.
To get started with Spring Boot and microservices, you will need to have the following software installed on your machine:
- Java 8 or higher
- An IDE such as Eclipse or IntelliJ IDEA
- Apache Maven, a build automation tool
Once you have these prerequisites set up, you can follow these steps to create a simple microservices application with Spring Boot:
Create a new Maven project in your IDE.
- 1 - Add the Spring Boot starter dependencies to the project’s pom.xml file. This will include the core Spring Boot libraries as well as any additional dependencies needed for your application.
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
-
2 - Create a main class that will be the entry point for your application. This class should be annotated with @SpringBootApplication and contain a main method that calls the SpringApplication.run method.
- 3 - Create a controller class to define the endpoints for your service. This class should be annotated with @RestController and contain methods annotated with @RequestMapping to handle HTTP requests.
- 4 - Define the business logic for your service in a separate service class. This class should contain the methods that will be called by the controller to perform the required tasks.
- 5 - Run the application by executing the main method in your main class.
That’s all there is to it! With just a few simple steps, you can create a microservices application with Spring Boot. Of course, a real-world application will likely be much more complex, but this basic outline will give you a good foundation to build upon.
Here is some sample code to illustrate the steps described above:
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
@RestController
@RequestMapping("/api")
public class MyController {
private final MyService myService;
public MyController(MyService myService) {
this.myService = myService;
}
@GetMapping("/greeting")
public String getGreeting() {
return myService.getGreeting();
}
}
@Service
public class MyService {
public String getGreeting() {
return "Hello, World!";
}
}
I hope this tutorial has given you a good understanding of how to use Spring Boot to build microservices applications. With its powerful set of features and ease of use, Spring Boot is a great choice for building scalable, reliable applications.