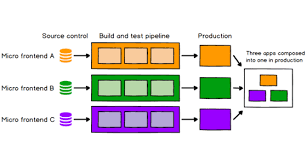
Microfrontends
Microfrontends is a term used to describe a modern architectural approach to building web applications where a single application is decomposed into smaller, self-contained components known as “microfrontends.” Each microfrontend is responsible for a specific feature or functionality within the overall application and is typically developed, deployed, and maintained by a separate team.
The main benefit of using microfrontends is that it allows teams to work on different parts of the application independently and at their own pace, without affecting the overall application. This can greatly improve the development process by reducing the risk of conflicts and making it easier to deploy new features and updates.
In this tutorial, we will walk through an example of how to build a simple web application using microfrontends. We will use React as our frontend framework, but the principles of microfrontends can be applied to any framework.
Prerequisites
Before we begin, make sure you have the following tools installed on your machine:
Node.js npm (comes with Node.js)
Setting up the project
First, create a new directory for your project and navigate to it in your terminal. Then, run the following command to initialize a new npm project:
npm init -y
This will create a package.json file in your project directory with default values.
Next, install React and the React Router library by running the following command:
npm install react react-router-dom
Creating the microfrontends In this tutorial, we will create two microfrontends: a homepage and a dashboard.
Create a new directory called homepage and navigate to it in your terminal. Then, run the following command to create a new React app:
npx create-react-app .
This will create a new React app in the homepage directory.
Next, create a new file called Homepage.js in the src directory and add the following code:
import React from 'react';
const Homepage = () => {
return (<div>
<h1>Homepage</h1>
<p>Welcome to the homepage microfrontend!</p>
</div>
);
};
export default Homepage;
This is a simple React component that displays a heading and a paragraph.
Next, create a new directory called dashboard and repeat the steps above to create a new React app. Then, create a new file called Dashboard.js in the src directory and add the following code:
import React from 'react';
const Dashboard = () => {
return (
<div>
<h1>Dashboard</h1>
<p>Welcome to the dashboard microfrontend!</p>
</div>
);
};
export default Dashboard;
This is another simple React component that displays a heading and a paragraph.
Integrating the microfrontends Now that we have our two microfrontends, we need to integrate them into a single web application.
Create a new directory called main-app and navigate to it in your terminal. Then, run the following command to create a new React app:
npx create-react-app .
Next, install the single-spa-react library by running the following command:
npm install single-spa-react
This library allows us to easily integrate multiple React applications as microfrontends into a single application.
Next, create a new file called main.js in the src directory and add the following code:
import * as singleSpa from 'single-spa';
import { loadApp } from 'single-spa-react';
const runScript = (url) => {
return new Promise((resolve, reject) => {
const script = document.createElement('script');
script.src = url;
script.onload = resolve;
script.onerror = reject;
document.head.appendChild(script);
});
};
const loadHomepage = async () => {
await runScript('http://localhost:3000/main.js');
return window.homepage.default;
};
const loadDashboard = async () => {
await runScript('http://localhost:3001/main.js');
return window.dashboard.default;
};
singleSpa.registerApplication(
'homepage',
loadHomepage,
(location) => location.pathname === '/'
);
singleSpa.registerApplication(
'dashboard',
loadDashboard,
(location) => location.pathname === '/dashboard'
);
singleSpa.start();
This code defines two functions, loadHomepage and loadDashboard, which are responsible for loading the homepage and dashboard microfrontends respectively. It also uses the single-spa library to register these microfrontends and specify the routes on which they should be mounted.
Next, update the App component in App.js to the following:
import React from 'react';
import { HashRouter, Route } from 'react-router-dom';
const App = () => {
return (
<HashRouter>
<Route exact path="/" render={() => <div>Homepage</div>} />
<Route exact path="/dashboard" render={() => <div>Dashboard</div>} />
</HashRouter>
);
};
export default App;
This is a simple routing component that defines two routes, one for the homepage and one for the dashboard.
Finally, update the index.js file to the following:
import React from 'react';
import { render } from 'react-dom';
import singleSpaReact from 'single-spa-react';
import App from './App';
const domElementGetter = () => document.getElementById('root');
const reactLifecycles = singleSpaReact({
React,
ReactDOM: ReactDOM,
rootComponent: App,
domElementGetter,
});
export const bootstrap = [reactLifecycles.bootstrap];
export const mount = [reactLifecycles.mount];
export const unmount = [reactLifecycles.unmount];
This code uses the single-spa-react library to define the lifecycle functions for the main application. These functions, bootstrap, mount, and unmount, are called by the single-spa library at different points in the application’s lifecycle.
Now that we have set up our main application, we need to build and serve the microfrontends.
First, navigate to the homepage directory and run the following command to build the microfrontend:
npm run build
This will create a build directory containing the compiled code for the microfrontend.
Next, start a local development server for the microfrontend by running the following command:
npm install -g serve
serve -s build
This will start a development server at http://localhost:3000 serving the compiled code for the homepage microfrontend.
Repeat these steps for the dashboard microfrontend, replacing 3000 with 3001 in the commands above.
Finally, navigate to the main-app directory and start the main application by running the following command:
npm start
This will start a development server at http://localhost:8080 serving the main application.
If everything is set up correctly, you should be able to navigate to http://localhost:8080 in your browser and see the homepage microfrontend, and navigate to http://localhost:8080/dashboard to see the dashboard microfrontend.
Conclusion In this tutorial, we have walked through an example of how to build a web application using microfrontends. By decomposing our application into smaller, self-contained components, we can improve the development process and make it easier to deploy new features and updates.
I hope this tutorial has been helpful! If you have any questions or comments, feel free to leave them below.