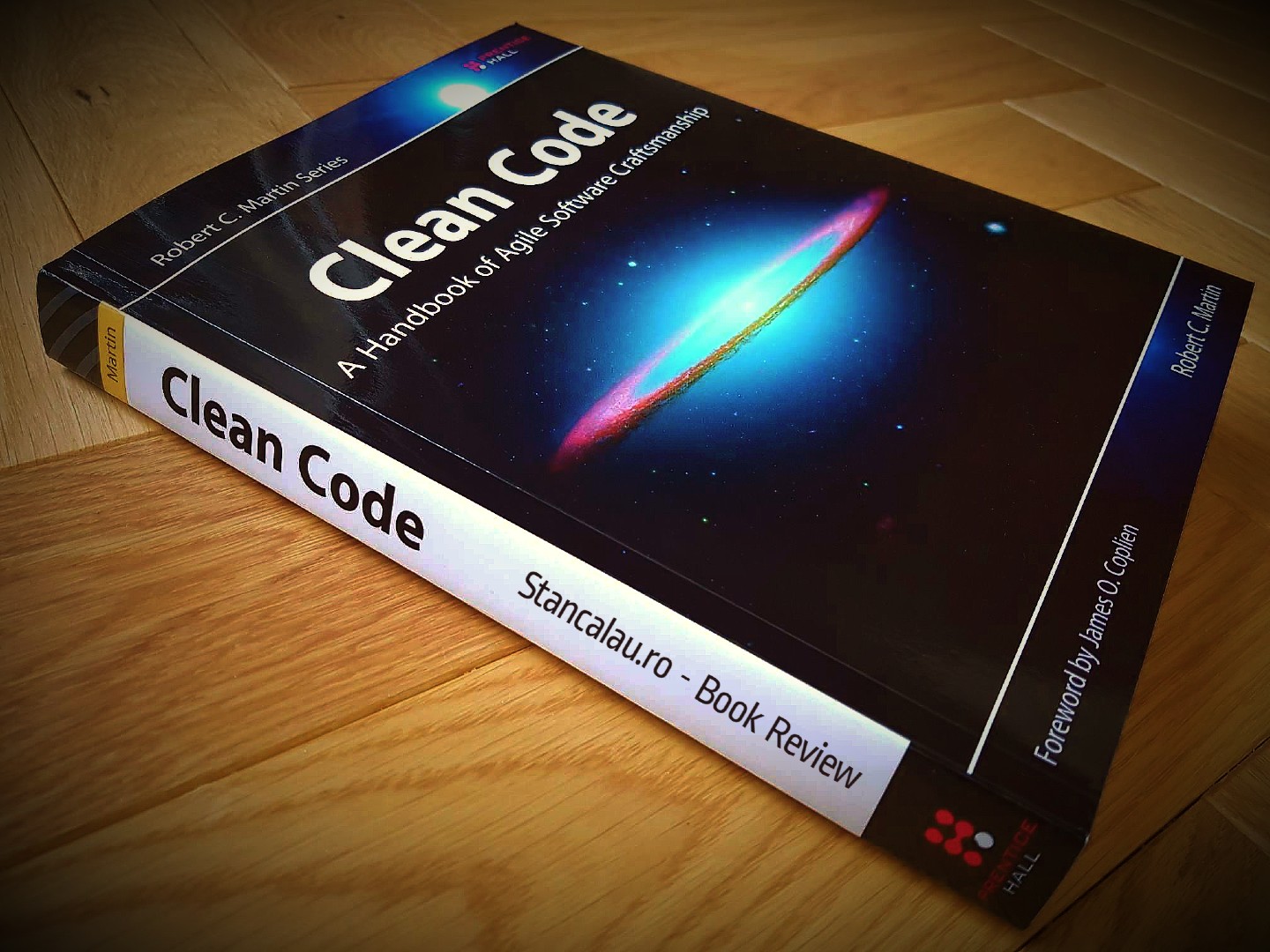
The Clean Code book
The Clean Code by Robert Martin is a classic book on software development best practices. It provides guidance on how to write code that is easy to understand, maintain, and extend. In this list, we’ll discuss ten key takeaways from the book that will help you write clean and effective code. These principles include the importance of using meaningful names, keeping functions small, using comments sparingly, following a consistent style, and more. By incorporating these principles into your work, you’ll be well on your way to writing code that is both functional and easy to read and understand.
-
Use meaningful names: Choose descriptive and unambiguous names for variables, functions, and other identifiers.
-
Keep functions small: Functions should ideally do one thing and do it well. Large functions are difficult to understand and maintain.
-
Use comments sparingly: Code should be self-explanatory and require minimal comments. If a piece of code is complex and requires a comment, it may be a sign that the code should be refactored.
-
Follow a consistent style: Establishing a consistent style for formatting, indentation, and naming conventions helps to improve readability and maintainability.
-
Write simple code: Avoid unnecessary complexity and try to keep code as simple as possible. This makes it easier to understand and maintain.
-
Avoid duplication: Duplicated code is more difficult to maintain and can introduce bugs. When you see duplication, try to refactor the code to eliminate it.
-
Keep code clean: Regularly review and refactor code to ensure it is readable, maintainable, and well-structured.
-
Use exception handling sparingly: Exception handling should be used only for exceptional situations, not as a way to control flow.
-
Test your code: Writing thorough unit tests helps to ensure that code is correct and maintainable.
-
Refactor mercilessly: Regularly review and refactor code to ensure it is clean and easy to understand. This will make it easier to maintain and extend over time.
-
Keep the codebase small: A smaller codebase is easier to understand and maintain. Try to avoid adding unnecessary features or code that doesn’t add value.
-
Use a version control system: A version control system, such as Git, allows you to track changes to your codebase and roll back to previous versions if needed. It also makes it easier to collaborate with others.
-
Write unit tests: Unit tests help to ensure that individual units of code are working correctly. They also make it easier to refactor code and make changes without breaking existing functionality.
-
Use design patterns: Design patterns are reusable solutions to common design problems. By using design patterns, you can create more flexible and maintainable code.
-
Follow SOLID principles: The SOLID principles are a set of guidelines for designing object-oriented software. They include principles such as the Single Responsibility Principle (SRP), which states that a class should have only one reason to change, and the Open/Closed Principle (OCP), which states that a class should be open for extension but closed for modification. Adhering to these principles can help you create more flexible and maintainable code.