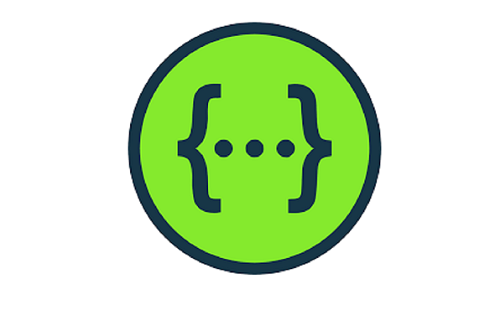
Swagger Editor
Introduction
Swagger (now known as the OpenAPI specification) is a popular tool for designing and documenting REST APIs. It provides a simple, yet powerful way to describe the structure and behavior of your APIs, allowing developers to easily understand and consume them. Swagger can be used with a variety of programming languages, including Java.
In this tutorial, we will learn how to use the Swagger/OpenAPI addons for Java to implement a REST API. We will start by setting up a basic project and installing the necessary dependencies. Then, we will design our API using the Swagger Editor and generate the server-side code using the Swagger Codegen tool. Finally, we will implement the API using the generated code as a starting point.
Prerequisites
To follow this tutorial, you will need:
A Java development environment, such as Eclipse or IntelliJ IDEA The Apache Maven build tool installed on your system Setting up the project
First, let’s create a new Maven project in our Java development environment. Make sure to select the “Web Application” archetype and specify a group ID and artifact ID for your project. This will create a basic project structure with a pom.xml file and a src/main/java directory.
Next, we need to install the following dependencies in the pom.xml file:
swagger-jaxrs: this is the core library that provides support for generating Swagger documentation from JAX-RS (Java API for RESTful Web Services) annotated classes. swagger-annotations: this is a library that provides additional annotations for describing the structure and behavior of your APIs. swagger-codegen-maven-plugin: this is a Maven plugin that generates server-side code based on a Swagger specification. Here is an example of how you can add these dependencies to the pom.xml file:
<project>
<dependencies>
<dependency>
<groupId>io.swagger</groupId>
<artifactId>swagger-jaxrs</artifactId>
<version>2.1.5</version>
</dependency>
<dependency>
<groupId>io.swagger</groupId>
<artifactId>swagger-annotations</artifactId>
<version>2.1.5</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>io.swagger</groupId>
<artifactId>swagger-codegen-maven-plugin</artifactId>
<version>2.4.7</version>
<executions>
<execution>
<goals>
<goal>generate</goal>
</goals>
<configuration>
<inputSpec>${project.basedir}/src/main/resources/swagger.yaml</inputSpec>
<language>java</language>
<library>jersey2</library>
<modelPackage>com.example.model</modelPackage>
<apiPackage>com.example.api</apiPackage>
<invokerPackage>com.example.invoker</invokerPackage>
<configOptions>
<interfaceOnly>true</interfaceOnly>
</configOptions>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
Designing the API with Swagger Editor
Now that we have set up the project and installed the necessary dependencies, we can start designing our API using the Swagger Editor. The Swagger Editor is a tool that allows you to design and document your APIs using the OpenAPI specification.
To start the Swagger Editor, run the following command in the root directory of your project:
Copy code java -jar swagger-editor.jar This will launch the Swagger Editor in your default web browser.
In the Swagger Editor, you can define the structure and behavior of your API by specifying the endpoints, HTTP methods, request and response payloads, and other details. The Swagger Editor provides a user-friendly interface and real-time feedback to help you design your API quickly and accurately.
Here is an example of a simple API definition in the Swagger Editor:
swagger: '2.0'
info:
title: Simple API
version: '1.0'
host: localhost:8080
basePath: /api
schemes:
- http
paths:
/greeting:
get:
summary: Returns a greeting
responses:
'200':
description: A greeting
schema:
type: string
This API definition specifies a single endpoint “/greeting” that can be accessed using the HTTP GET method. When this endpoint is called, it returns a string containing a greeting.
Generating the server-side code with Swagger Codegen
Once you have designed your API using the Swagger Editor, you can use the Swagger Codegen tool to generate the server-side code. The Swagger Codegen tool is a command-line tool that reads a Swagger specification and generates code in a variety of languages.
To generate the server-side code for your API, run the following command in the root directory of your project:
mvn clean package
This will invoke the Swagger Codegen Maven plugin and generate the server-side code based on the Swagger specification stored in the src/main/resources/swagger.yaml file. The generated code will be stored in the target/generated-sources directory, and will include the following:
-
Model classes: These are Java classes that represent the request and response payloads of your API. They are annotated with the JAXB (Java Architecture for XML Binding) annotations, which allow them to be serialized and deserialized from XML or JSON.
-
API interface: This is a Java interface that defines the methods of your API. It is annotated with the JAX-RS annotations, which allow it to be implemented as a RESTful web service.
-
Invoker class: This is a Java class that implements the API interface and provides the actual implementation of your API.
Implementing the API
Now that we have generated the server-side code, we can start implementing our API. To do this, we need to create a new Java class that extends the Invoker class and overrides the methods defined in the API interface.
Here is an example of how you can implement the API:
import com.example.api.SimpleApi;
import com.example.model.Greeting;
import javax.ws.rs.core.Response;
public class SimpleApiImpl extends SimpleApi {
@Override
public Response getGreeting() {
Greeting greeting = new Greeting();
greeting.setMessage("Hello, World!");
return Response.ok().entity(greeting).build();
}
}
In this example, the SimpleApiImpl class extends the SimpleApi class (which is generated by Swagger Codegen) and overrides the getGreeting method. This method returns a Response object containing a Greeting object with a message field set to “Hello, World!”.
To deploy your API, you will need to create a JAX-RS application class and register the SimpleApiImpl class as a resource. Here is an example of how you can do this:
import javax.ws.rs.ApplicationPath;
import javax.ws.rs.core.Application;
import java.util.HashSet;
import java.util.Set;
@ApplicationPath("/api")
public class SimpleApplication extends Application {
@Override
public Set<Class<?>> getClasses() {
Set<Class<?>> resources = new HashSet<>();
resources.add(SimpleApiImpl.class);
return resources;
}
}
In this example, the SimpleApplication class extends the Application class and overrides the getClasses method. This method returns a Set containing the SimpleApiImpl class, which is registered as a resource at the “/api” path.
To deploy the API, you will need to run a web server that supports JAX-RS, such as Apache Tomcat or Jetty. You will also need to include the necessary dependencies in your project, such as the jersey-server library.
Conclusion
In this tutorial, we have learned how to use the Swagger/OpenAPI addons for Java to implement a REST API. We started by setting up a basic project and installing the necessary dependencies. Then, we designed our API using the Swagger Editor and generated the server-side code using the Swagger Codegen tool. Finally, we implemented the API using the generated code as a starting point.
Using Swagger/OpenAPI can greatly simplify the process of designing and implementing APIs, as it provides a standard and easy-to-use way to describe the structure and behavior of your APIs. I hope this tutorial has been helpful and has given you a good understanding of how to use Swagger/OpenAPI with Java.