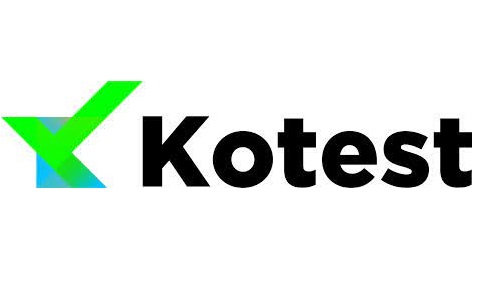
Unit testing with Kotlin
Unit testing is a critical part of the software development process as it helps ensure that individual units of code are working correctly and as expected. In this tutorial, we will learn how to write unit tests using Kotest, a powerful and flexible testing framework for Kotlin.
Before we begin, it’s important to understand the basics of unit testing. A unit test is a piece of code that tests a small, specific unit of functionality in your application. This unit is usually a single function or method, but it could also be a group of related functions or a class. The goal of a unit test is to validate that this unit of code is functioning correctly and producing the expected output.
To get started with Kotest, you will need to have the Kotlin programming language and the Kotest library installed on your machine. You can install Kotlin through the official website or using a package manager such as SDKMAN or Homebrew. To install Kotest, you will need to include the following dependency in your build.gradle file:
testImplementation("io.kotest:kotest-framework-engine:4.3.3")
Once Kotest is installed, you can begin writing your unit tests. Kotest uses a syntax similar to JUnit, so if you are familiar with JUnit, you will feel right at home. To write a unit test, you will need to create a new class and annotate it with the @Test annotation. Here’s an example of a simple unit test in Kotest:
@Test
fun testAddition() {
val result = 2 + 2
assertEquals(4, result)
}
In this example, we are testing a simple addition function. We use the assertEquals method to verify that the result of the addition is equal to the expected value of 4. If the test passes, it means that the addition function is working correctly. If the test fails, it means that there is a bug in the function that needs to be fixed.
Kotest also provides a wide range of additional features and functionality to help you write more comprehensive and effective unit tests. For example, you can use the @BeforeTest and @AfterTest annotations to specify code that should be run before and after each test. You can also use the @Ignore annotation to temporarily disable a test if it is not ready to be run.
In addition to these core features, Kotest also offers a number of additional test types and assertions to help you write more robust and flexible tests. For example, you can use the expect function to test for specific exceptions, or the assertTrue and assertFalse functions to test for boolean values. You can also use the assertFailsWith function to test that a specific exception is thrown.
Overall, Kotest is a powerful and flexible testing framework that makes it easy to write and run unit tests in Kotlin. With its intuitive syntax and rich feature set, Kotest is a valuable tool for ensuring the quality and reliability of your code. Whether you are a beginner or an experienced developer, Kotest is an essential tool for writing reliable and effective unit tests.