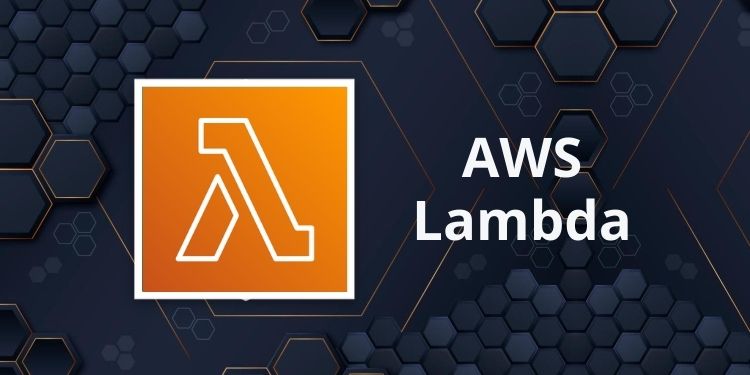
How to Create a Serverless App Using AWS Lambdas
Creating a serverless app using AWS Lambdas is a quick and easy way to deploy your code without the hassle of managing servers. This method is perfect for applications that have a high amount of traffic and need to scale up or down quickly.
In this article, we will show you how to create a serverless app using AWS Lambdas and the AWS Cloud Development Kit ( CDK) with code examples in TypeScript.
First, let’s start by setting up our development environment. You will need to have the following tools installed:
- AWS CLI
- AWS CDK
- Node.js and NPM
- TypeScript
Once you have all these tools installed, we can proceed with creating our serverless app.
Step 1: Create a new AWS CDK project
To create a new AWS CDK project, run the following command in your terminal:
cdk init --language=typescript
This will create a new directory with the default CDK project structure.
Step 2: Create an AWS Lambda function
To create an AWS Lambda function, we need to define a new class that extends the Lambda class from the aws-cdk-lib package.
import {Lambda} from 'aws-cdk-lib';
class MyLambdaFunction extends Lambda {
constructor(scope: Construct, id: string, props?: LambdaProps) {
super(scope, id, props);
// Your code here...
}
}
In the constructor, we pass in the scope and id of the Lambda function, as well as any optional props such as the runtime, memory size, and environment variables.
Next, we can define the code for our Lambda function. This can be done by adding a new Code object to the props parameter.
import {Lambda, Code} from 'aws-cdk-lib';
class MyLambdaFunction extends Lambda {
constructor(scope: Construct, id: string, props?: LambdaProps) {
super(scope, id, {
...props,
code: Code.fromAsset('src/my-lambda-function/index.ts')
});
}
}
In this example, we are loading the code for our Lambda function from a local file called index.ts in the src/my-lambda-function directory.
Step 3: Create an AWS API Gateway
Now that we have our Lambda function, we can create an AWS API Gateway to trigger our function. To do this, we need to define a new class that extends the RestApi class from the aws-cdk-lib package.
import {RestApi} from 'aws-cdk-lib';
class MyApiGateway extends RestApi {
constructor(scope: Construct, id: string, props?: RestApiProps) {
super(scope, id, props);
// Your code here...
}
}
In the constructor, we pass in the scope and id of the API Gateway, as well as any optional props such as the default stage name and stage variables.
Next, we can create a new resource on our
API Gateway and attach our Lambda function to it.
import {RestApi, LambdaIntegration} from 'aws-cdk-lib';
class MyApiGateway extends RestApi {
constructor(scope: Construct, id: string, props?: RestApiProps) {
super(scope, id, props);
// Create a new resource on the API Gateway
const resource = this.root.addResource('my-resource');
// Attach the Lambda function to the resource
resource.addMethod('GET', new LambdaIntegration(myLambdaFunction));
}
}
In this example, we are creating a new resource called my-resource and attaching our myLambdaFunction to it. When a GET request is made to this resource, our Lambda function will be triggered.
Step 4: Deploy the serverless app
Now that we have our Lambda function and API Gateway set up, we can deploy our serverless app to AWS. To do this, we need to create a new class that extends the Stack class from the aws-cdk-lib package.
import {Stack} from 'aws-cdk-lib';
class MyServerlessApp extends Stack {
constructor(scope: Construct, id: string, props?: StackProps) {
super(scope, id, props);
// Your code here...
}
}
In the constructor, we pass in the scope and id of the stack, as well as any optional props such as the stack name and environment.
Next, we can add our Lambda function and API Gateway to the stack.
import {Stack, Lambda, RestApi} from 'aws-cdk-lib';
class MyServerlessApp extends Stack {
constructor(scope: Construct, id: string, props?: StackProps) {
super(scope, id, props);
// Create a new Lambda function
const myLambdaFunction = new MyLambdaFunction(this, 'my-lambda-function');
// Create a new API Gateway
const myApiGateway = new MyApiGateway(this, 'my-api-gateway');
}
}
Finally, we can deploy our serverless app by running the cdk deploy command in our terminal.
cdk
deploy
This will deploy our stack to AWS, creating all the necessary resources such as the Lambda function, API Gateway, and IAM roles.
Conclusion
In this article, we showed you how to create a serverless app using AWS Lambdas and the AWS Cloud Development Kit (CDK) with code examples in TypeScript. We covered the steps to set up our development environment, create a Lambda function and API Gateway, and deploy our app to AWS.
By using serverless technologies like AWS Lambdas, you can easily deploy your code without worrying about managing servers and scaling up or down to handle high traffic. With the CDK, you can write your app in a familiar language like TypeScript and deploy it with just a few simple commands.