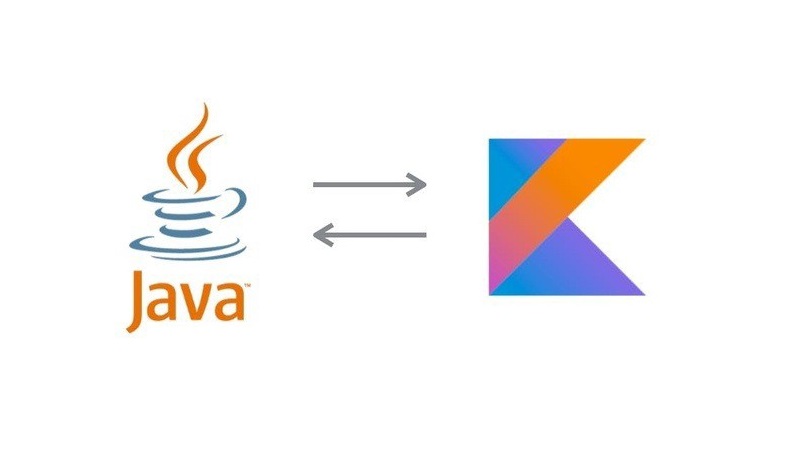
From Java to Kotlin
As a Java developer, the decision to move to a new programming language can be daunting. However, the switch to Kotlin, a modern and concise language, can provide numerous benefits and enhance your development experience.
First and foremost, Kotlin is fully interoperable with Java, meaning that you can use Kotlin code within a Java project and vice versa. This allows for a smooth transition and the ability to incorporate Kotlin gradually into your existing codebase.
In terms of language features, Kotlin offers a more concise syntax compared to Java. For example, null safety is built into the language, eliminating the potential for null pointer exceptions. This is achieved through the use of nullable and non-nullable types, as well as safe calls and the Elvis operator.
Additionally, Kotlin supports higher-order functions and lambdas, allowing for more concise and readable code when working with collections. For instance, the following Java code can be simplified in Kotlin using a higher-order function:
Java:
List<String> names=Arrays.asList("John","Jane","Jack");
for(String name:names){
System.out.println(name);
}
Kotlin:
val names = listOf("John", "Jane", "Jack")
names.forEach { println(it) }
Kotlin also introduces the concept of data classes, which automatically generate boilerplate code such as constructors, equals, hashCode, and toString methods. This allows for a more efficient and clean codebase. For example:
Java:
public class User {
private String name;
private int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
@Override
public boolean equals(Object o) {
if (this == o)
return true;
if (o == null || getClass() != o.getClass())
return false;
User user = (User) o;
return age == user.age && Objects.equals(name, user.name);
}
@Override
public int hashCode() {
return Objects.hash(name, age);
}
@Override
public String toString() {
return "User{" + "name='" + name + '\'' + ", age=" + age + '}';
}
}
Kotlin:
data class User(val name: String, val age: Int)
Overall, the switch to Kotlin can greatly improve the efficiency and readability of your code. The interoperability with Java allows for a seamless transition, and the added language features provide a more concise and effective development experience.
Another advantage of Kotlin is its ability to leverage the existing Java frameworks and libraries. As Kotlin is fully interoperable with Java, you can easily use any Java libraries in your Kotlin code. Additionally, Kotlin has its own set of libraries and frameworks, such as the Kotlinx.serialization library for efficient and flexible serialization, and the Ktor framework for building asynchronous server applications.
Moreover, the Kotlin community is growing and thriving, with numerous resources and support available for developers. The language is backed by JetBrains, the company behind popular IDEs such as IntelliJ IDEA and Android Studio, which provide built-in support for Kotlin.
In terms of adoption, Kotlin is gaining popularity in the industry. According to the 2019 Developer Survey by Stack Overflow, Kotlin is the fourth most loved programming language and the sixth most wanted language among developers. Major companies such as Pinterest, Netflix, and Trello have already adopted Kotlin in their tech stacks.
However, there are a few cautions to keep in mind when using Kotlin interop with Java.
First, Kotlin has a different type system than Java, which can lead to some differences in behavior. For example, Kotlin has nullable types, which allows a variable to either contain a value or be null. In contrast, Java does not have nullable types, and it is common to use a special value (such as -1 or null) to indicate that a variable does not have a valid value. This can lead to confusion when calling Kotlin code from Java, as the Java code may not be able to handle null values properly.
Another issue to be aware of is that Kotlin code can sometimes generate additional bytecode that is not present in the equivalent Java code. This can lead to increased memory usage and slower performance when running Kotlin code on the JVM. This is because Kotlin adds additional metadata to its bytecode to support features such as null safety and data classes, which are not present in Java.
Finally, there are some differences in syntax and naming conventions between Kotlin and Java, which can make it more difficult to read and understand code that mixes the two languages. For example, Kotlin uses camelCase for naming variables and functions, while Java uses camelCase with initial caps. Additionally, Kotlin has more concise syntax for certain constructs, such as properties and data classes, which can make it more difficult to understand for Java developers who are not familiar with Kotlin.
While Kotlin interop with Java is generally seamless and allows developers to use the best of both languages, it is important to be aware of these potential issues and to test and optimize code as necessary to ensure that it runs smoothly in a mixed-language environment.
Here are some additional hints and best practices to keep in mind when using Kotlin interop with Java:
-
Use the
@JvmOverloads
annotation to generate multiple overloads of a Kotlin function in Java. This can be helpful when calling a Kotlin function from Java and you want to avoid having to specify default parameter values in the function call. -
Use the
@JvmName
annotation to specify a different name for a Kotlin function in the generated Java bytecode. This can be useful when you want to avoid naming conflicts or simply prefer a different naming style in the Java version of your code. -
Use the
@JvmField
annotation to expose a Kotlin property as a public field in the generated Java bytecode. This can be useful when you want to access the property directly from Java without having to call a getter or setter function. -
Use the
@JvmStatic
annotation to generate a static member in the Java version of a Kotlin class or object. This can be helpful when you want to call a Kotlin function or access a Kotlin property from a static context in Java. -
Be mindful of the nullability of Kotlin types when calling Kotlin code from Java. Make sure to check for null values and handle them appropriately, or use nullable types in Kotlin to indicate that a value may be null.
-
Use the
lateinit
keyword in Kotlin to declare a non-null property that is initialized later. This can be helpful when you need to initialize a property from Java code, as you can avoid having to use a nullable type. -
Use the
@Throws
annotation to declare that a Kotlin function can throw an exception in Java. This can be helpful when calling a Kotlin function from Java and you want to handle the exception in a try-catch block.
By following these best practices and being aware of the potential issues, you can effectively use Kotlin interop with Java to take advantage of the strengths of both languages and build robust and efficient applications.
Overall, the switch to Kotlin can greatly benefit Java developers in terms of language features, interoperability, and community support. As a modern and concise language, Kotlin provides numerous advantages and enhances the development experience.