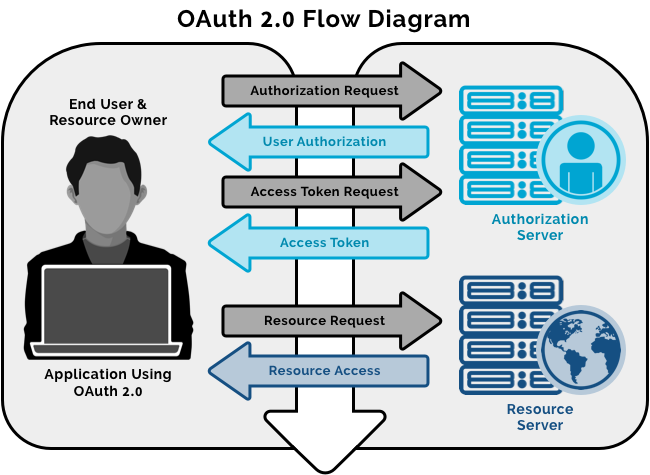
Brief look into oAuth2
OAuth2 is an authorization framework that enables applications to obtain limited access to user accounts on an HTTP service, such as Facebook, GitHub, and Google. It works by allowing users to authorize third-party applications to access their account information without revealing their password.
JWT (JSON Web Token) is a compact, URL-safe means of representing claims to be transferred between two parties. The claims in a JWT are encoded as a JSON object that is digitally signed using JSON Web Signature (JWS) and/or encrypted using JSON Web Encryption (JWE).
In this tutorial, we will demonstrate how to use OAuth2 and JWT with a client built with ReactJS and TypeScript.
Setting up the project
To get started, create a new ReactJS project with TypeScript by running the following command:
npx create-react-app my-app --template typescript
Next, we will install the necessary dependencies for handling OAuth2 and JWT. Run the following command to install the axios and jwt-decode packages:
npm install axios jwt-decode
Handling OAuth2 authentication
First, we need to set up an OAuth2 client on the service that we want to authenticate with. This usually involves creating a new application and obtaining a client ID and client secret.
Next, we will create a login component that redirects the user to the service’s authorization URL. This URL should include the client ID, the callback URL (which should be set to the URL of the login component), and a list of scopes that the application is requesting access to.
Here is an example of a login component that redirects the user to the GitHub authorization URL:
import React, {useEffect} from 'react';
const Login: React.FC = () => {
useEffect(() => {
const clientId = 'YOUR_CLIENT_ID';
const redirectUri = encodeURIComponent('http://localhost:3000/login');
const scope = 'user:email';
window.location.href = `https://github.com/login/oauth/authorize?client_id=${clientId}&redirect_uri=${redirectUri}&scope=${scope}`;
}, []);
return null;
};
export default Login;
Once the user has authorized the application, they will be redirected back to the callback URL with an authorization code in the query string. We can then use this code to request an access token from the service’s token endpoint.
Here is an example of how to request an access token using the axios library:
import axios from 'axios';
const clientId = 'YOUR_CLIENT_ID';
const clientSecret = 'YOUR_CLIENT_SECRET';
const code = 'AUTHORIZATION_CODE';
const redirectUri = 'http://localhost:3000/login';
axios.post('https://github.com/login/oauth/access_token', {
client_id: clientId,
client_secret: clientSecret,
code,
redirect_uri: redirectUri,
}, {
headers: {
Accept: 'application/json',
},
}).then(response => {
console.log(response.data);
});
The response from the token endpoint will include an access token, which we can use to authenticate API requests on behalf of the user.
To make API requests, we can use the axios
library and set the Authorization
header to the access token, like this:
import axios from 'axios';
const accessToken = 'YOUR_ACCESS_TOKEN';
axios.get('https://api.github.com/user', {
headers: {
Authorization: `Bearer ${accessToken}`,
},
}).then(response => {
console.log(response.data);
});
Handling JWT authentication
JWT is often used as an alternative to OAuth2 for authenticating API requests. In this case, the client sends a JWT in the Authorization header of the API request to prove its authenticity.
To create a JWT, we will need to install the jsonwebtoken package:
npm install jsonwebtoken
Next, we will create a function that generates a JWT using the jsonwebtoken library. This function should take in the user’s ID and a secret key, and return the JWT as a string:
import jwt from 'jsonwebtoken';
const secret = 'YOUR_SECRET_KEY';
const generateJWT = (userId: string) => {
return jwt.sign({userId}, secret, {expiresIn: '1h'});
};
We can then use the generateJWT function to create a JWT for a user and send it in the Authorization header of an API request:
import axios from 'axios';
const userId = 'USER_ID';
const jwt = generateJWT(userId);
axios.get('https://api.example.com/protected', {
headers: {
Authorization: `Bearer ${jwt}`,
},
}).then(response => {
console.log(response.data);
});
On the server side, we can use the jsonwebtoken library to verify the authenticity of the JWT and extract the user’s ID from the payload.
import jwt from 'jsonwebtoken';
const secret = 'YOUR_SECRET_KEY';
const verifyJWT = (jwt: string) => {
return jwt.verify(jwt, secret);
};
const userId = verifyJWT(jwt).userId;
Conclusion
In this tutorial, we have demonstrated how to use OAuth2 and JWT to authenticate API requests with a client built with ReactJS and TypeScript. OAuth2 is a widely used authorization framework that enables users to authorize third-party applications to access their account information, while JWT is a compact, URL-safe means of representing claims that can be used to authenticate API requests. Both OAuth2 and JWT are powerful tools for securing APIs and enabling third-party integrations.