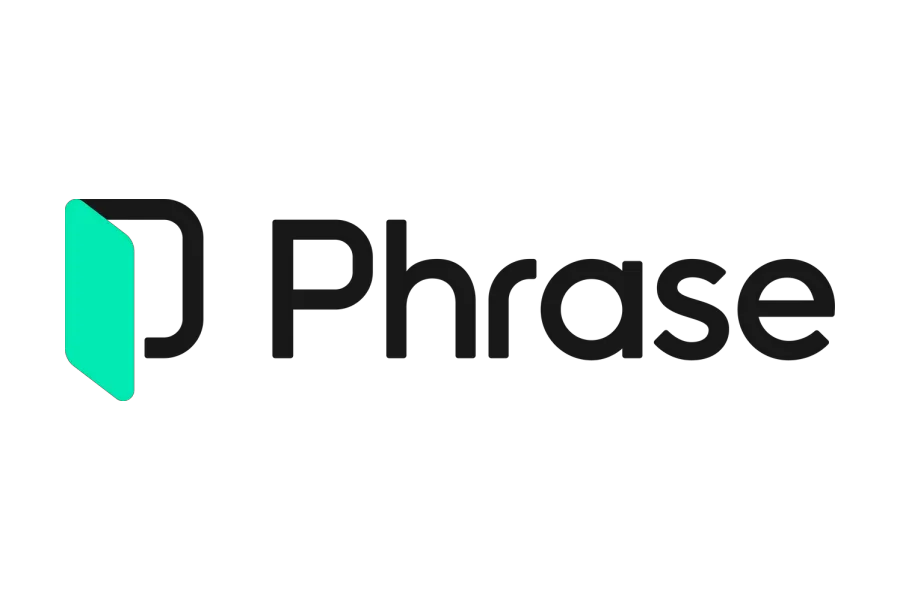
Phrase the translation service
Phrase is a powerful translation management platform that allows developers to easily localize their applications in multiple languages. One of the key features of Phrase.com is its integration with various programming languages and frameworks, including ReactJS. In this tutorial, we will show you how to implement a small ReactJS application using Phrase.com.
Before we begin, make sure you have the following prerequisites:
- A Phrase.com account: You can sign up for a free trial at https://phrase.com/.
- Node.js and npm: You will need Node.js and npm (which comes with Node.js) installed on your machine to install ReactJS and the Phrase.com library. You can download Node.js and npm from https://nodejs.org/.
- A ReactJS application: If you don’t already have a ReactJS application, you can use the create-react-app command to create a new one. Simply run the following command in your terminal:
npm install -g create-react-app
create-react-app my-app
cd my-app
With these prerequisites in place, we can now proceed to integrate Phrase.com into our ReactJS application.
Install the Phrase.com library for ReactJS: To install the Phrase.com library for ReactJS, run the following command in your terminal:
npm install @phrase/react
Initialize the Phrase.com library: In your ReactJS application, import the init function from the Phrase.com library and call it with your Phrase.com API key. You can find your API key in your Phrase.com account settings.
import {init} from '@phrase/react';
init({
apiKey: 'your-api-key-here'
});
Translate your React components: To translate a React component, simply wrap it with the Translate component from the Phrase.com library and specify the translation key for the component. The Translate component will automatically fetch the translated text from Phrase.com and render it in the correct language. For example, consider the following React component:
import {Translate} from '@phrase/react';
function Hello() {
return (
<div>
<h1>Hello, world!</h1>
</div>
);
}
To translate this component, simply wrap it with the Translate component and specify a translation key:
import {Translate} from '@phrase/react';
function Hello() {
return (
<Translate key="hello">
<div>
<h1>Hello, world!</h1>
</div>
</Translate>
);
}
Add translations to Phrase.com: To add translations to Phrase.com, log in to your Phrase.com account and create a new project. Then, click on the “Translations” tab and enter the translations for each language you want to support. That’s it! With these simple steps, you can easily add localization to your ReactJS application using Phrase.com.
I hope this tutorial was helpful. If you have any questions or need further assistance, don’t hesitate to ask.